REST API FOR AMAZON DYNAMODB CRUD OPERATIONS USING AWS LAMBDA
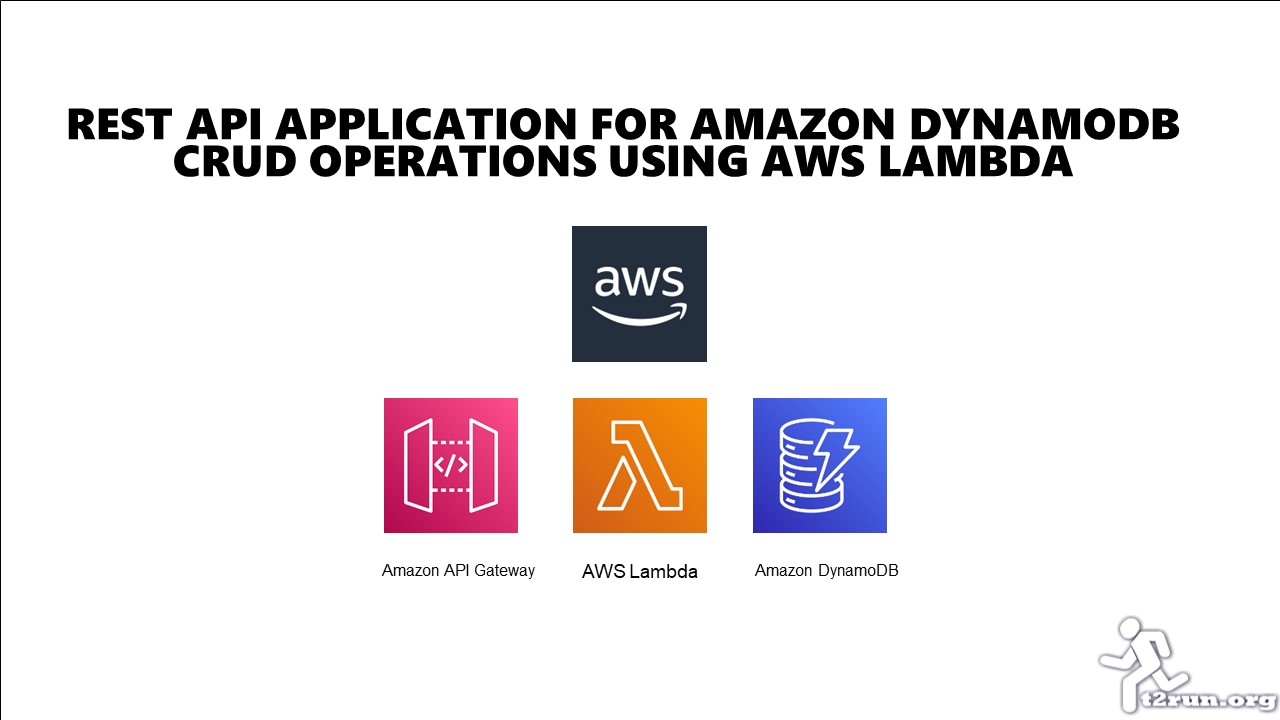
This tutorial helps you to build a REST API Application using Amazon API Gateway, DynamoDB and AWS Lambda.
Architecture Diagram
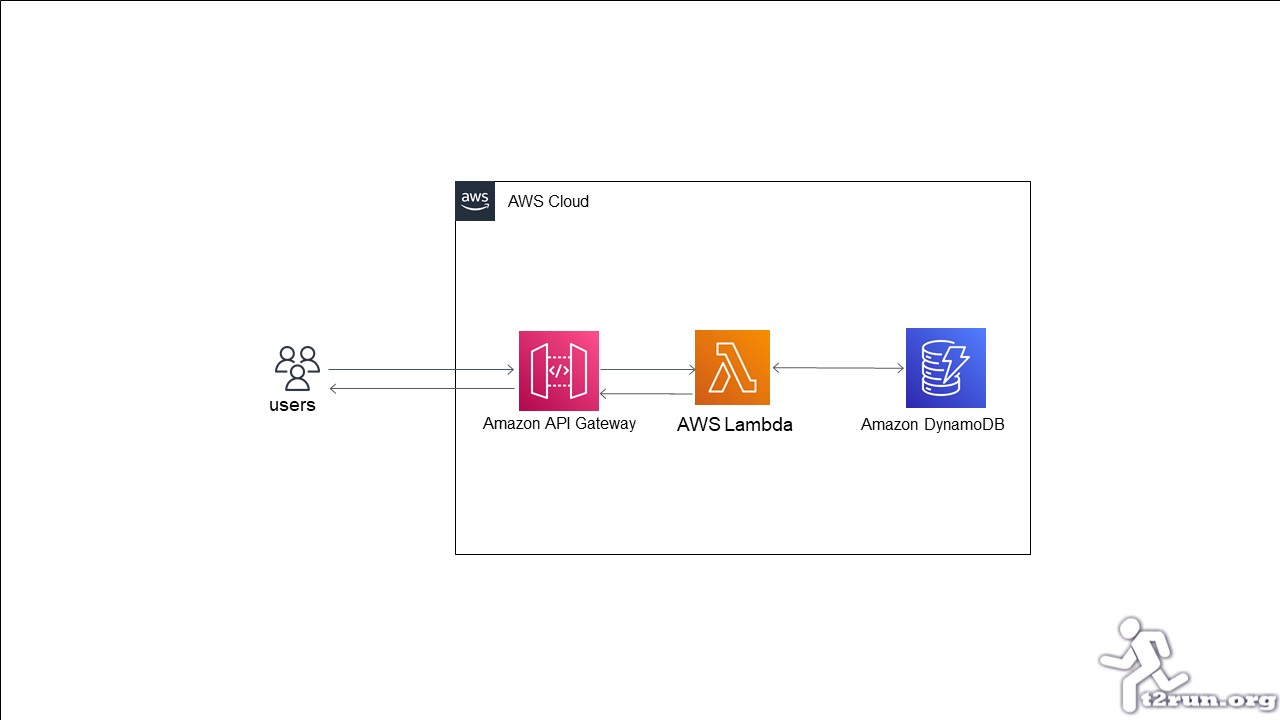
API Specification:
GET https://base-url/users/{userId}
DELETE https://base-url/users/{userId}
POST https://base-url/users/
request body:
{
"userId": "",
"firstName": "",
"lastName": ""
}
PUT https://base-url/users/
request body:
{
"userId": "",
"firstName": "",
"lastName": ""
}
How to Create Amazon DynamoDB?
Table Details:
Partition Key: userIdNo Sort Key
DynamoDB json:
{
"userId": {
"S": ""
},
"firstName": {
"S": ""
},
"lastName": {
"S": ""
}
}
DynamoDB Table Item's
userId | FistHName | LastName | |
---|---|---|---|
1 | WD001 | Rick | Grimes |
2 | DC0013 | joker | joker |
3 | WD002 | Maggie | Greene |
> Complete quide to create a Amazon DynamoDB
AWS Lambda Design
APIGateway Proxy request handler
func handler(ctx context.Context, request events.APIGatewayProxyRequest) (events.APIGatewayProxyResponse, error) {
switch request.HTTPMethod {
case "GET":
// Implementation for DynamoDB GET request (Read Operation)
case "POST":
// Implementation for POST DynamoDB request (Create Operation)
case "PUT":
// Implementation for PUT DynamoDB request (Update Operation)
case "DELETE":
// Implementation for DELETE DynamoDB request (Delete Operation)
default:
}
}
Input and Output of the handler function
func handler(ctx context.Context, request events.APIGatewayProxyRequest) (events.APIGatewayProxyResponse, error) {
}
Input Struct
// APIGatewayProxyRequest contains data coming from the API Gateway proxy
type APIGatewayProxyRequest struct {
Resource string `json:"resource"` // The resource path defined in API Gateway
Path string `json:"path"` // The url path for the caller
HTTPMethod string `json:"httpMethod"`
Headers map[string]string `json:"headers"`
MultiValueHeaders map[string][]string `json:"multiValueHeaders"`
QueryStringParameters map[string]string `json:"queryStringParameters"`
MultiValueQueryStringParameters map[string][]string `json:"multiValueQueryStringParameters"`
PathParameters map[string]string `json:"pathParameters"`
StageVariables map[string]string `json:"stageVariables"`
RequestContext APIGatewayProxyRequestContext `json:"requestContext"`
Body string `json:"body"`
IsBase64Encoded bool `json:"isBase64Encoded,omitempty"`
}
Output Struct
// APIGatewayProxyResponse configures the response to be returned by API Gateway for the request
type APIGatewayProxyResponse struct {
StatusCode int `json:"statusCode"`
Headers map[string]string `json:"headers"`
MultiValueHeaders map[string][]string `json:"multiValueHeaders"`
Body string `json:"body"`
IsBase64Encoded bool `json:"isBase64Encoded,omitempty"`
}
> Implementation of CRUD Operations
Building Code and Creating build Zip for Lambda
## To build for Linux for windows
### cmd
```cmd
set GOOS=linux
go build -o main main.go
```
### powershell
```powershell
$env:GOOS = "linux"
$env:CGO_ENABLED = "0"
$env:GOARCH = "amd64"
go build -o main main.go
```
## To create ZIP
### Get lambda zip tool
```
go.exe get -u github.com/aws/aws-lambda-go/cmd/build-lambda-zip
```
### To ZIP
#### cmd
``` cmd
%USERPROFILE%\Go\bin\build-lambda-zip.exe -output main.zip main
```
### powershell
``` powershell
~\Go\Bin\build-lambda-zip.exe -output main.zip main
```
How to Create AWS Lambda?
Using ConsoleSteps:
- Search and select AWS Lambda.
- Select Create Function Menu.
- Fill the details .
- In the Code tab, select upload file.
- Select the Build Zip file and upload.
- Edit the Runtime settings
- Update the handler function name from hello to main (main module function name which is
the entry point of code).
> Fill Function Name > Runtime
> Execution Role (Here I had created a execution rule with DynamoDB full access with name dynamoCrud)
Test the API from Lambda
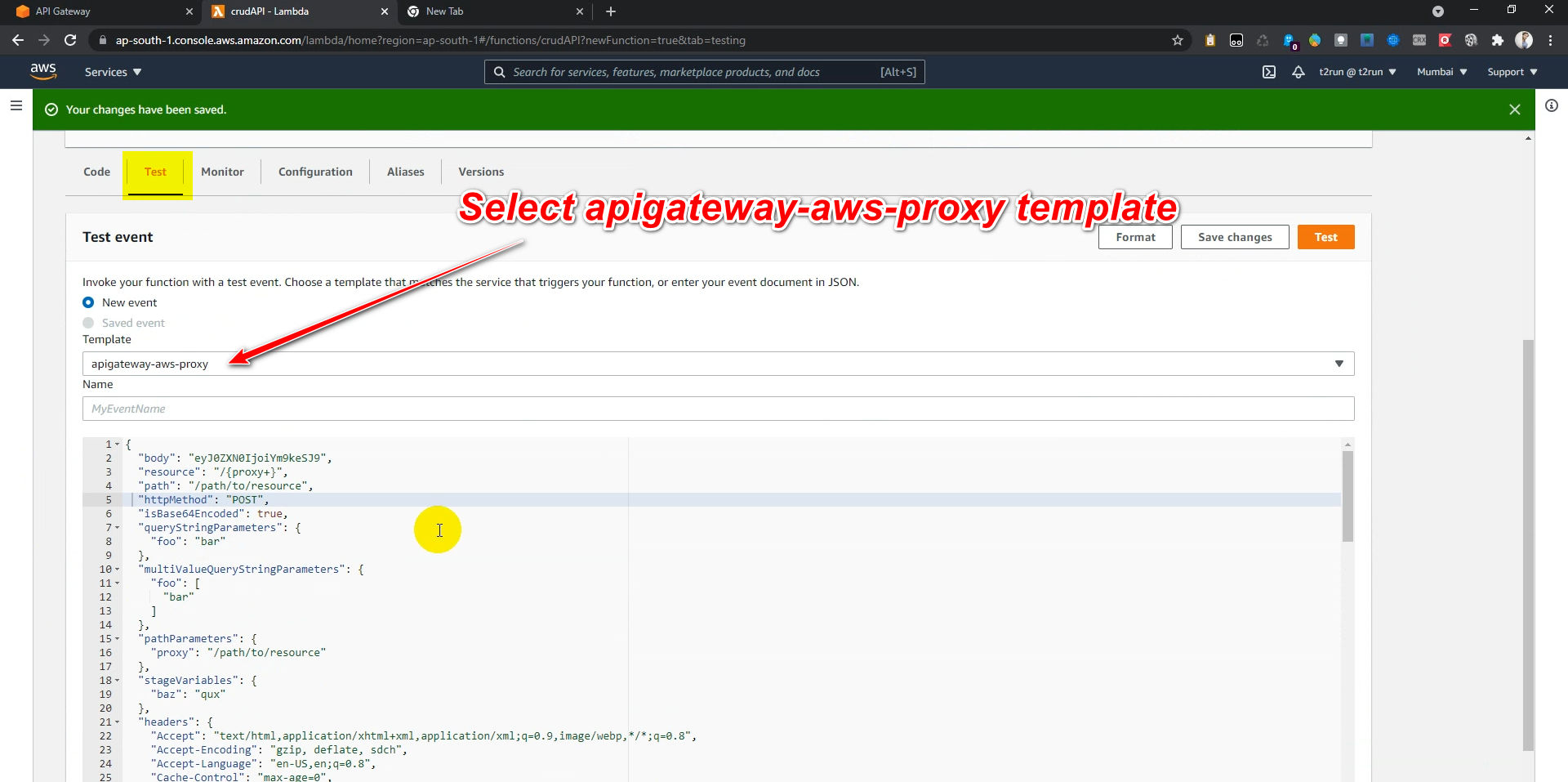
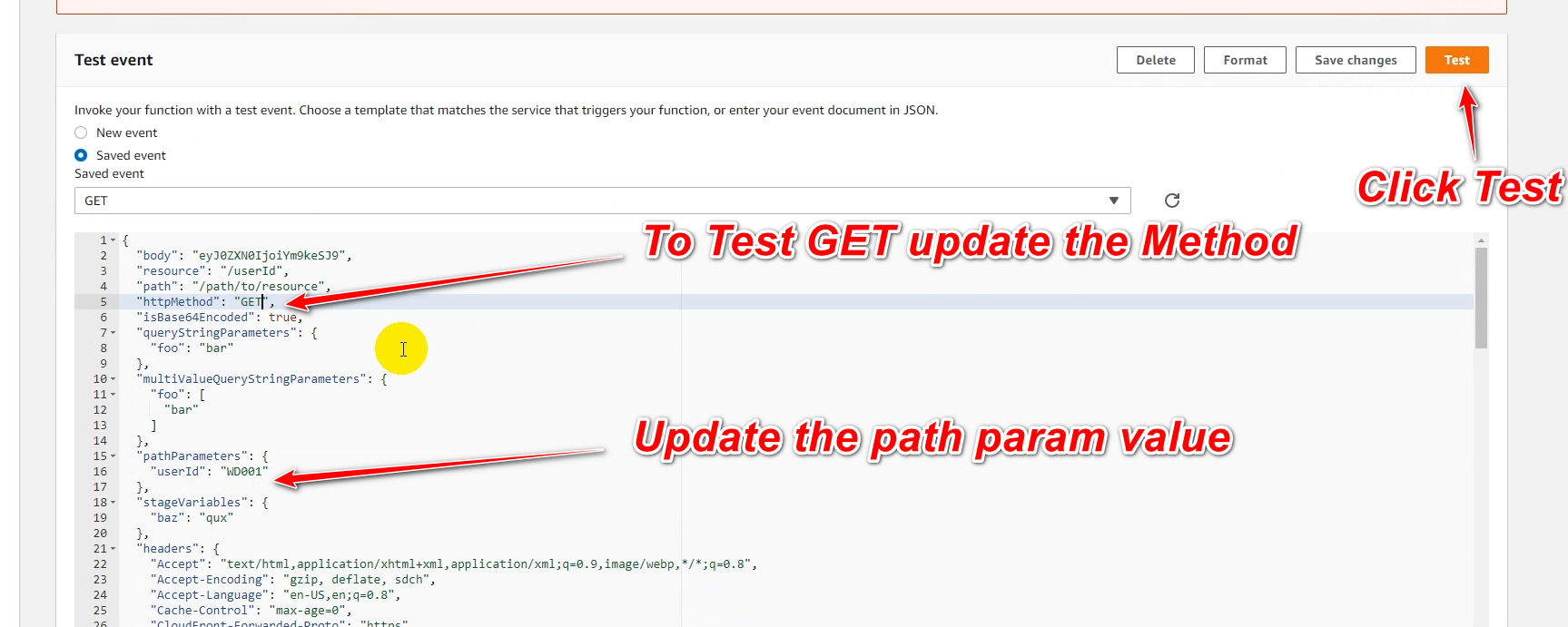
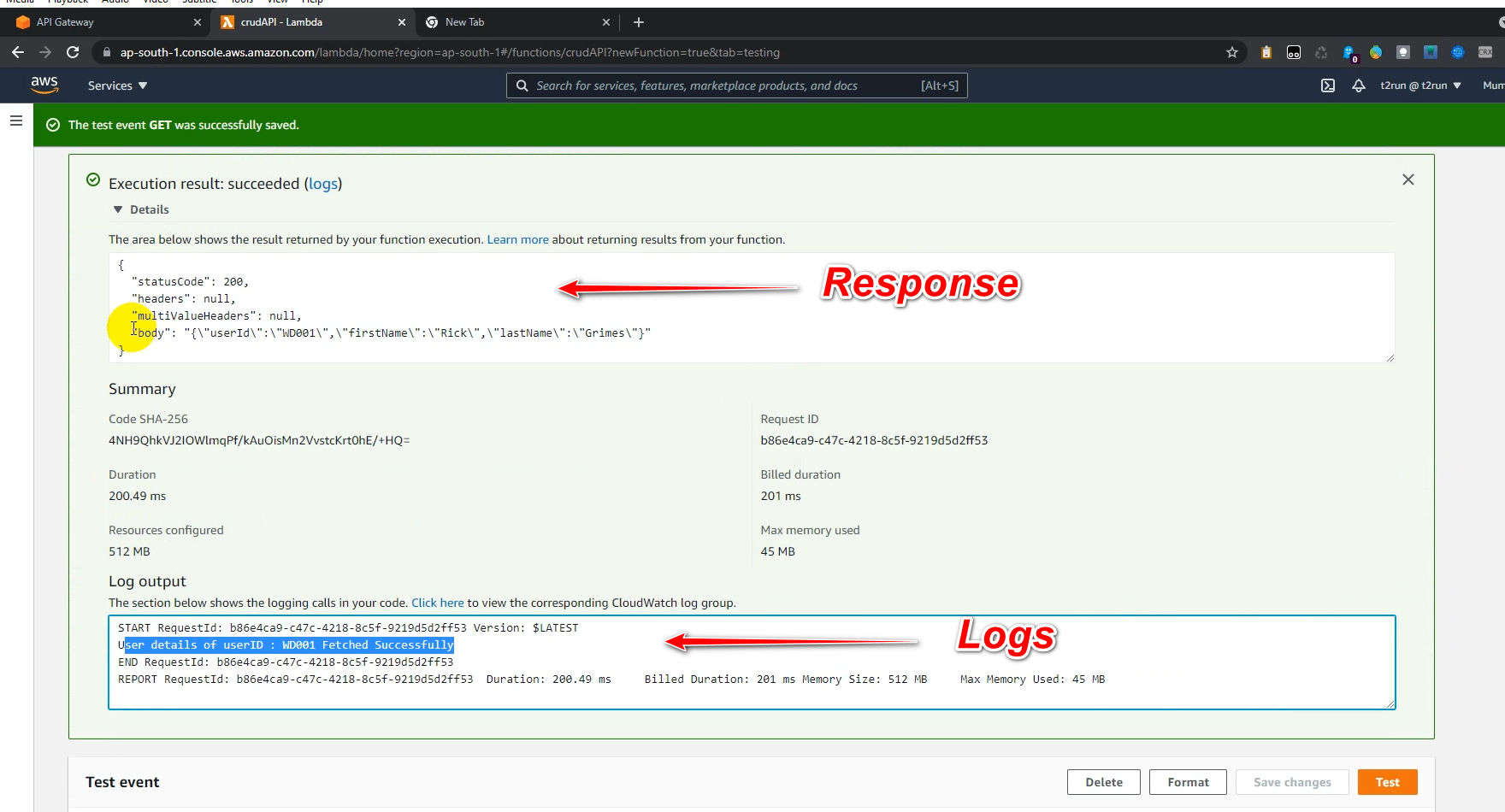
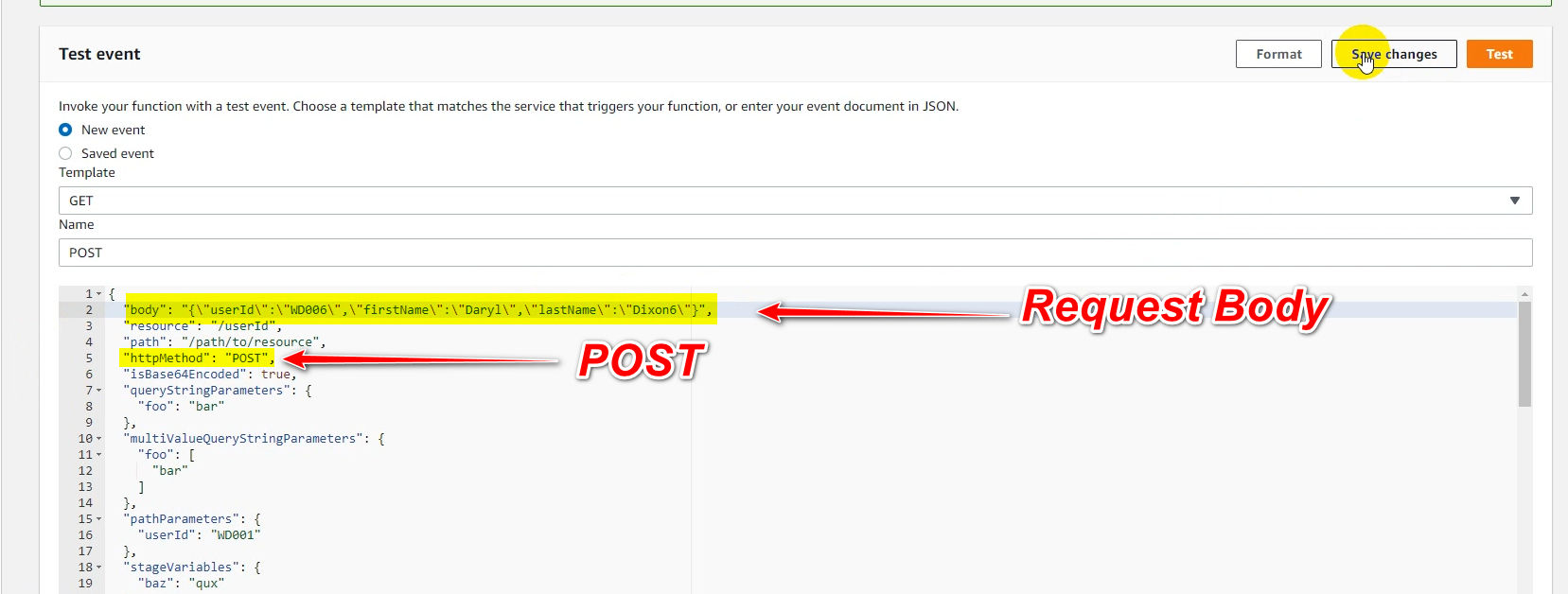
Create REST API using Amazon API Gateway
Using ConsoleSteps:
- Search and select Amazon API Gateway.
- Choose Protocol as REST, Fill API Name name and click Create API.
- Create Resource.
- Fill the Resource name for users.
- Fill the Resource name as path parameter for the userId.
- Create Methods.
- Create a GET method for the resource users.
- Similarly Create POST PUT DELETE Methods for Resources.
Integrate Amazon API Gateway with AWS Lambda
- Select the method
- Enable Proxy Integration
- Select Target Lambda and Save
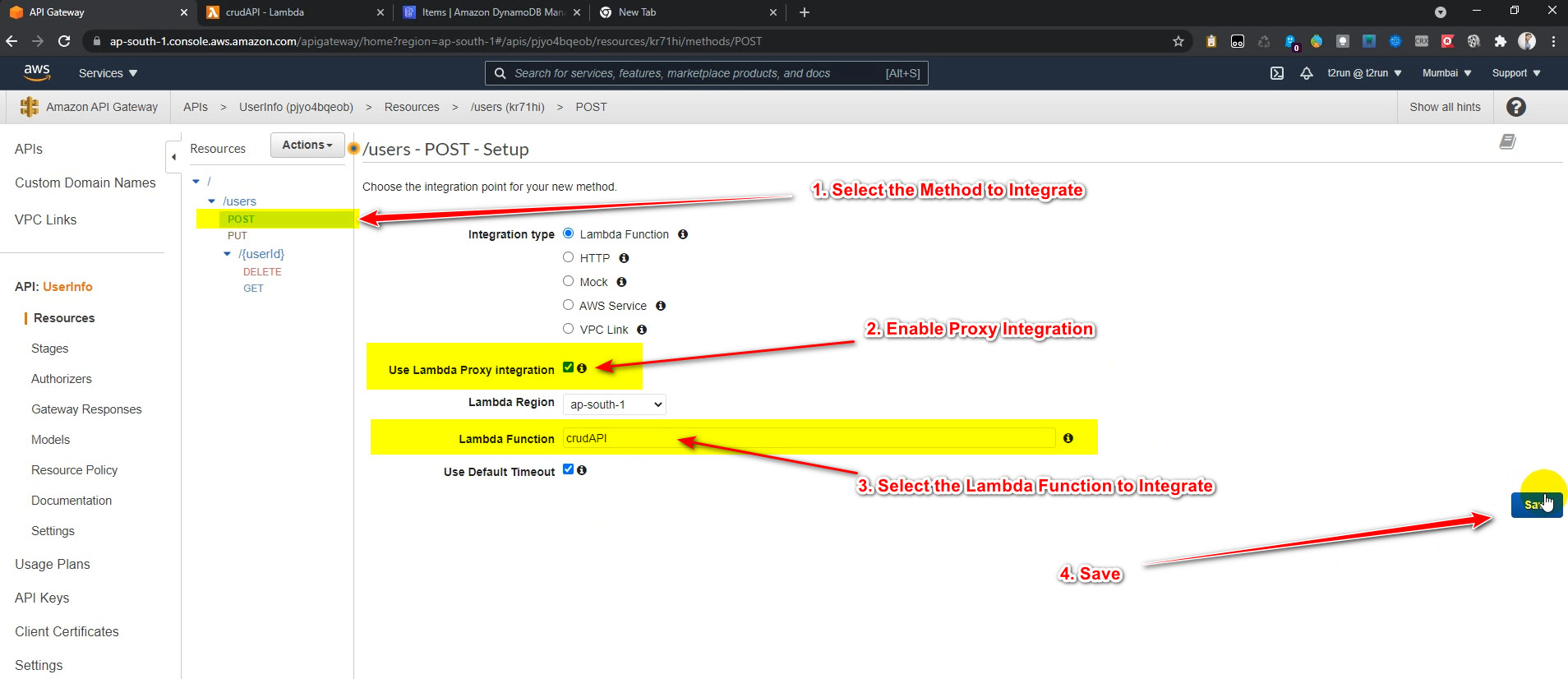
Test the API using Postman
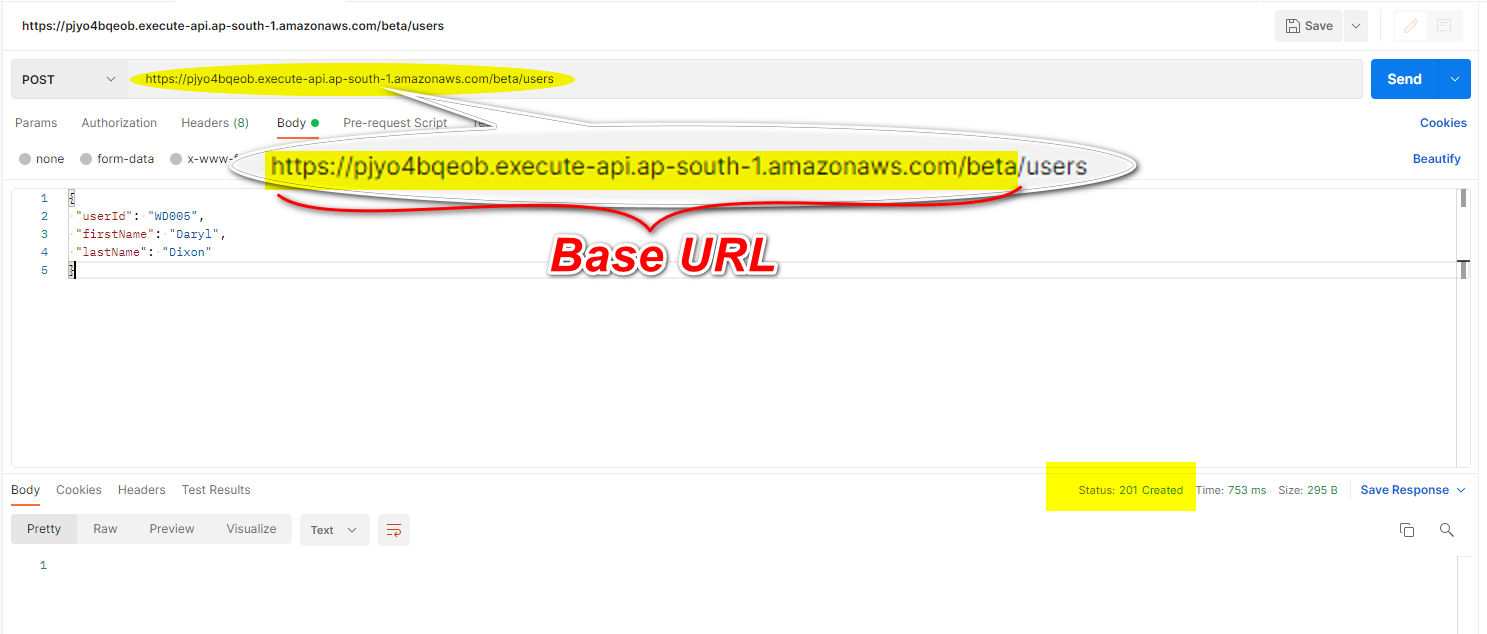
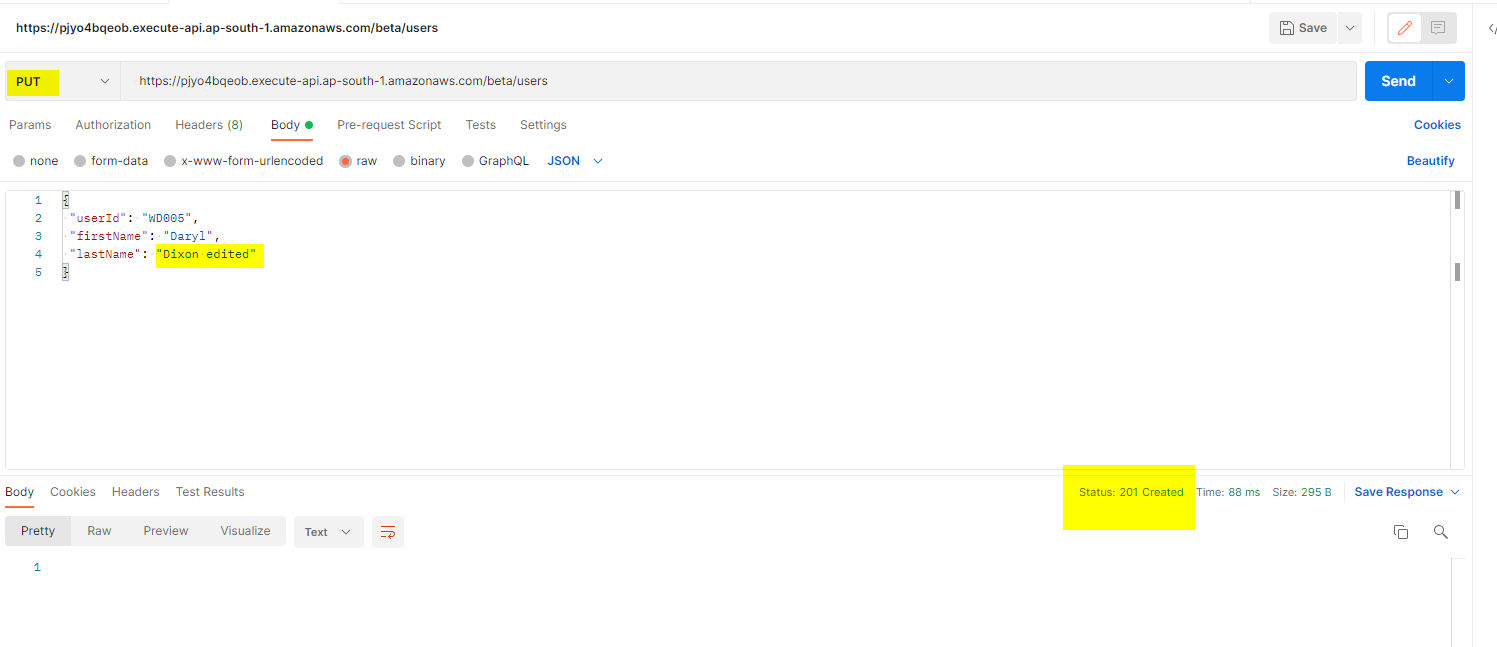
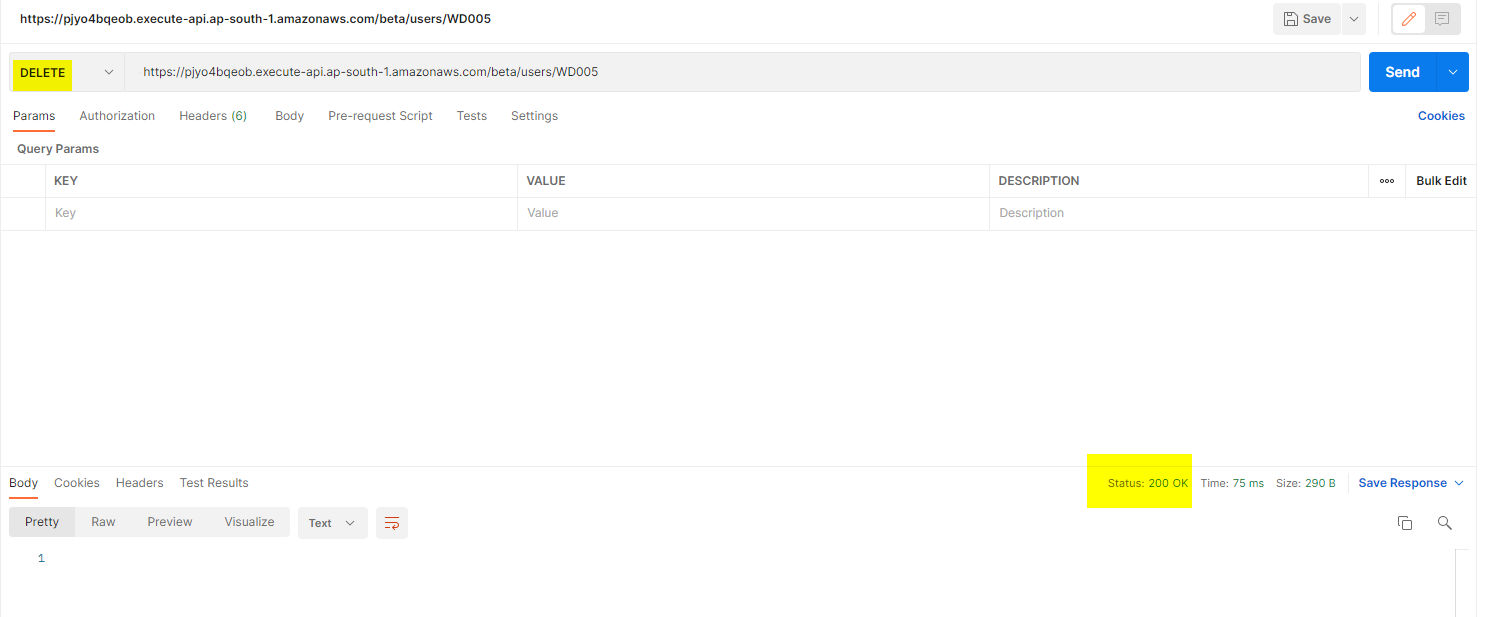
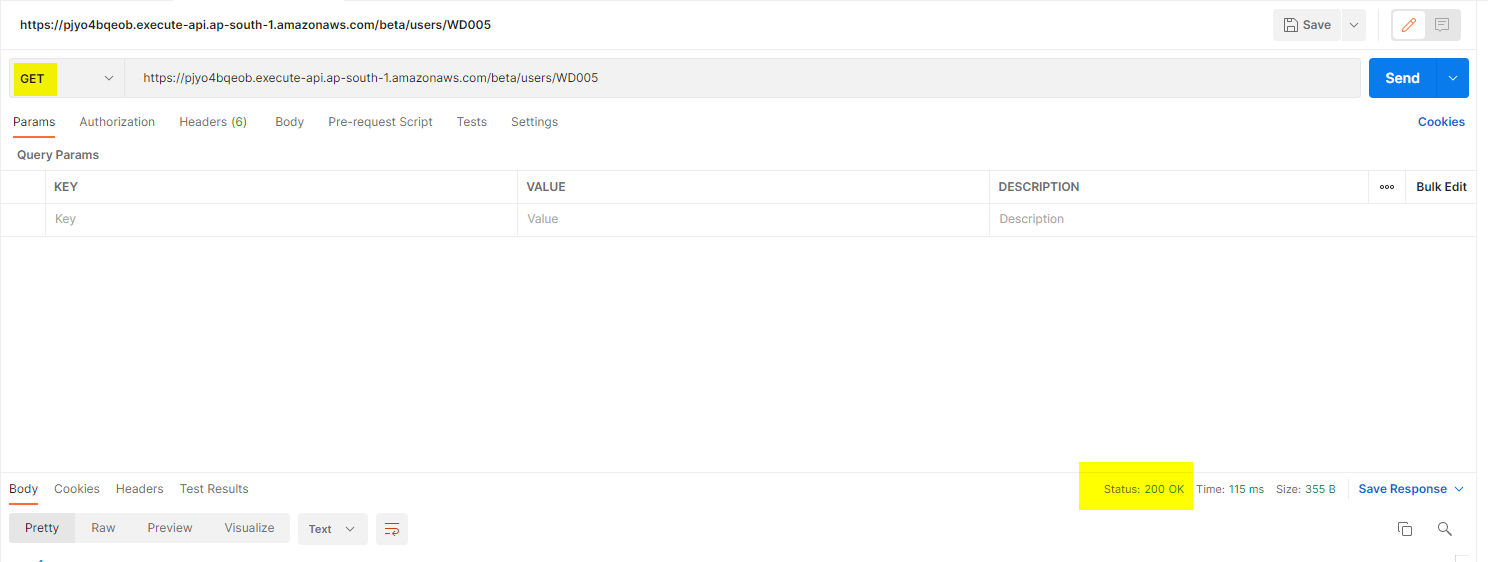
Conclusion:
We Successfully created a CRUD API Application using Amazon API Gateway, AWS Lambda and Amazon
DynamoDB.
We explored the AWS Lambda; How to create, build, zip and deploy a Go Application.
We created a Amazon API Gateway and Integrated the API with Lambda for the CRUD
Implementation.
We also learned how to create a Amazon DynamoDB and to Create an Item, Read an Item, Update
an Item and Delete an Item programmatically.
To Secure the API,We can modify the application to support the API with JWT Token authentication
or Lambda Authorizer.
Complete Code:
Fork Now GitHubReferences:
[1] golang-package[2] golang-handler
[3] Go AWS SDK
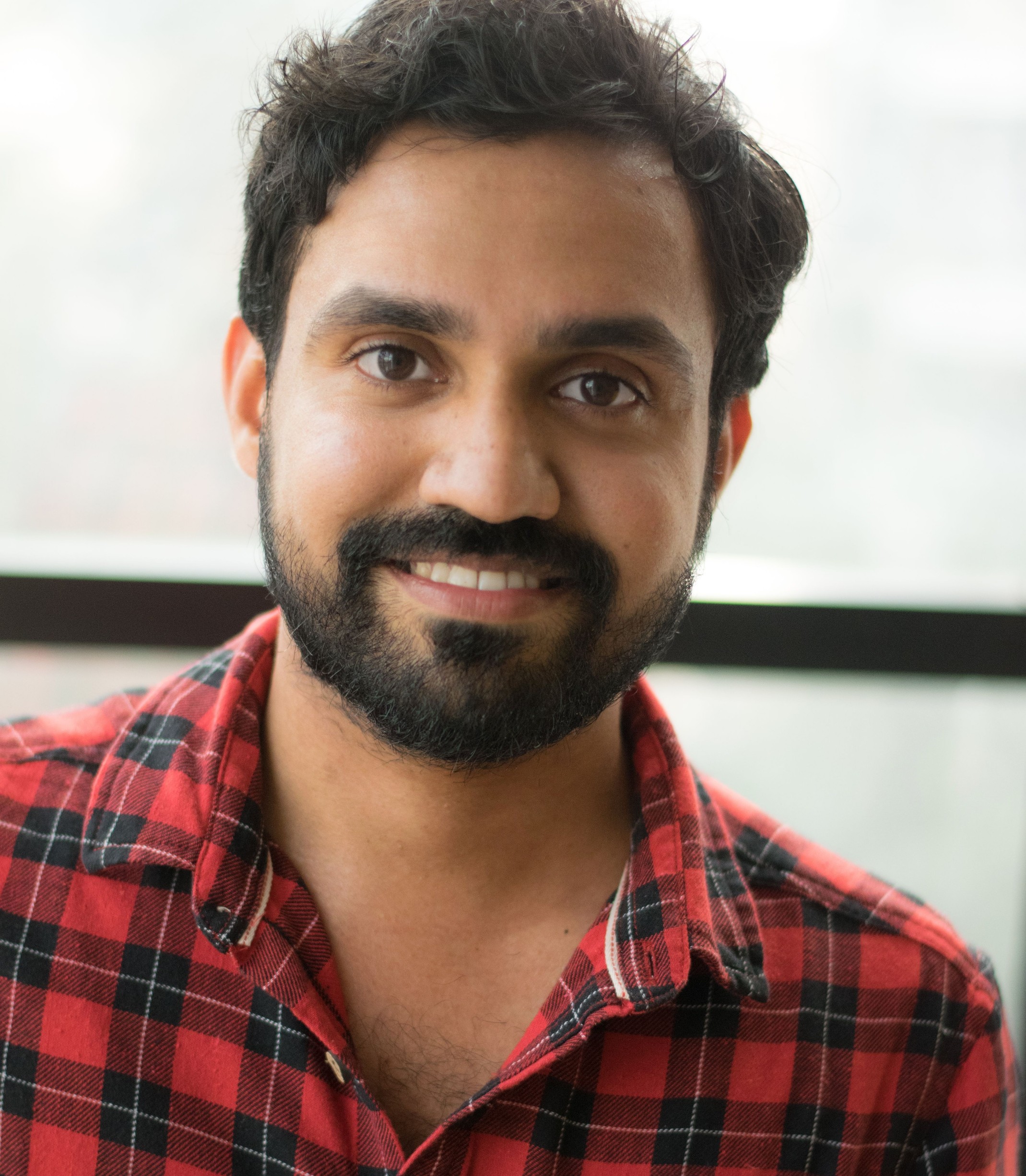
Written by
Arun N
Full stack developer. Go |5x AWS Certified | Angular
AWS CERTIFIED DEVELOPER ASSOCIATE | SOLUTION ARCHITECT | DATABASE – SPECIALTY | DATA ANALYTICS – SPECIALTY| MACHINE LEARNING – SPECIALTY